How to Make a Raspberry Pi Python Web Server
Because of its small footprint, energy efficiency, and reasonable computing power, the Raspberry Pi makes an excellent server. You can spin up a Raspberry Pi media server using the likes of Plex, Emby, and OpenMediaVault, or a web server running WordPress, Drupal, Apache, or NGINX. Similarly, you can make a Python web server with a Raspberry Pi and Flask. Learn how to build a Raspberry Py Python web server!
What is Python?
Python is a programming language. It's used for creating web applications and desktop apps alike. A general programming language, Python remains one of the top programming languages for beginners. Combined with Flask, a Python microframework, you can make your own Python-powered web server.
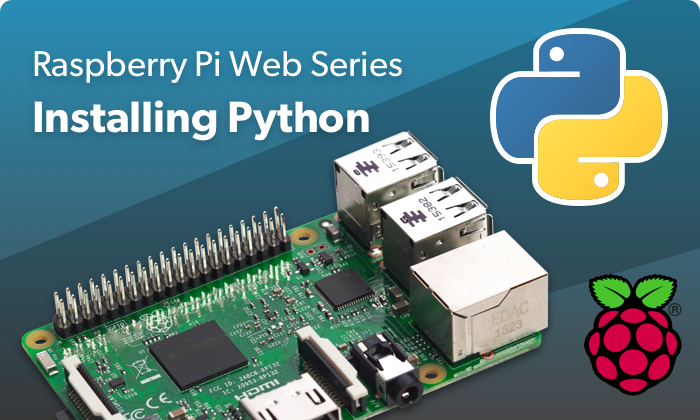
What you'll need for a Raspberry Pi Python web server:
- Raspberry Pi board (Raspberry Pi 4 recommended)
- Power supply
- microSD card
- Case (optional, recommended)
- Linux distribution (distro)
- Peripherals (keyboard, mouse)
- Python, Flask, pip
Total cost: $35+ USD. Chances are you may have most of the components for this project at home. You will need a Raspberry Pi which you can snag for around $35 standalone or opt for a kit with Pi, case, microSD card, and power supply. All software and your operating system (OS) are free.
How to Make a Raspberry Pi Python Web Server
First off, open a new terminal (CTRL + ALT + T) and install Flask with:
sudo apt-get install python3-flask
Next, make a fresh directory for this project:
mkdir pythonwebapp
Now change directories into this directory:
cd pythonwebapp
Under the menu, open Python. Using File > New file open up a new window, and save it as app.py within the pythonwebapp project folder you just made.
Then, add some sample code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return 'Hello world'
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0')
Hit CTRL + S to save, and back in a terminal window run:
python3 app.py
This executes the web server. If all went properly, you should see something similar to:
Running on http://0.0.0.0:5000/
Restarting with reloader
In a browser, head to http://127.0.0.1:5000/
and you should see a screen with the words "Hellow world." You may also use http://localhost:5000/
.
With Python and Flask installed, you may add routes. For instance, open your app.py file and add a sample route:
@app.route('/cupofmoe')
def cupofmoe():
return 'Raspberry Pie!'
What specifically you put here will vary. Save that file, and head to http://127.0.0.1:5000/cupofmoe
and you'll see the text you entered after "return."
For even more functionality, try adding HTML templates to your Raspberry Pi Python server. In a command line, navigate into your pythonwebapp
folder:
cd pythonwebapp
Then, create a templates directory:
mkdir templates
Open a text editor like Leafpad and enter your HTML code:
<html>
<body>
<h1>Hello world!</h1>
</body>
</html>
Save that as index.html under the templates directory under the pythonwebapp
directory.
Next, head to your app.py
file and edit the first line so that it imports the render_template
function:
from flask import Flask, render_template
When that's complete, edit the index view so that you're returning the HTML template you've created:
@app.route('/')
def index():
return render_template('index.html')
Save the file, and reload http://127.0.0.1:5000/ and you'll see whatever text was in your HTML template.
Want to jazz up your Python web server even more? Add some CSS! Navigate into your pythonwebapp
directory:
cd pythonwebapp
Make a directory named static:
mkdir static
Open a text editor like Leafpad, and make a style.css
file in your static directory. In this file, add some basic CSS such as:
body {
background: green;
color: black;
}
If you're feeling more creative, you can use hex codes instead of just color names. When you're finished, save the file. Head to your index.html file and add the CSS by creating a head tag with a link tag pointing to your stylesheet:
<html>
<head>
<link rel="stylesheet" href='/static/style.css' />
</head>
<body>
<h1>Hello world!</h1>
</body>
</html>
Save that HTML file, refresh your web page, and you should see the same text but with updated coloring.
How to Build a Raspberry Pi Python Web Server: Final Thoughts
Overall, a Python web server with Flask running on a Raspberry Pi is ridiculously simple to create. It's easier than spinning up a Drupal or WordPress server, and about on par with making an NGINX server. A Python-based Raspberry Pi server may be as complex or barebones as you like. Using HTML and CSS, you may spice up your site, and it's easy to add new routes.
What are you running on your Raspberry Pi?
Leave your feedback...