Arduino Tft Interfacing
About the project
By using this color TFT LCD shield we can show characters, strings, button interfacing, bitmap images, etc on the color TFT LCD
Project info
Difficulty: Moderate
Estimated time: 1 hour
License: GNU General Public License, version 3 or later (GPL3+)
Items used in this project
Story
Intro: Arduino TFT Interfacing
TFT touchscreens are the amazing graphical interface which can be used with microcontrollers such as Atmel, PIC, STM, as it has a wide color range, and good graphical ability and a good mapping of pixels.
Today, we are going to Interface 2.4 inch TFT LCD Shield with Arduino.
This shield is for Arduino UNO, but I'll teach how to use it with Arduino Mega for a very logical reason, the "Program Memory".
By using this color TFT LCD shield we can show characters, strings, button interfacing, bitmap images, etc on the color TFT LCD.
Step 1: Hardware and Software Requirements
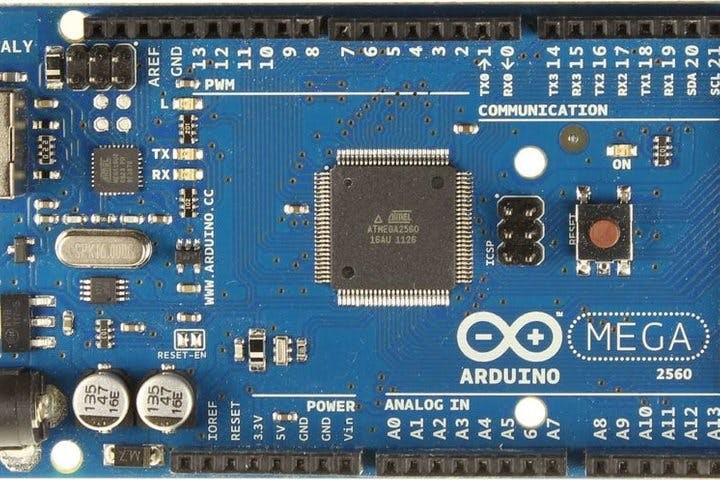
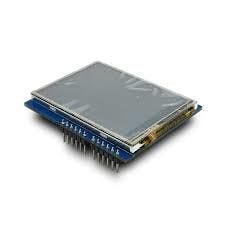
To make the shield interface with the Arduino mega, we need HARDWARE :
• Arduino mega
• TFT 2.4/2.8/3.2 inch LCD
• USB Cable
SOFTWARE :
• Arduino IDE
• UTFT Library / spfd5408 Library
The Shield is originally made for the Arduino UNO boards, which can be used with Arduino mega.
There is two main issue while using it with the Arduino UNO: "Storage memory" and pins usage.
It is difficult to use the unused pins which are available on UNO, whereas it is better with Arduino MEGA since we have more I/O pins left.
In the next step, I'll show how to edit the UTFT library to use the TFT shield
Step 2: Tweaking UTFT Lib
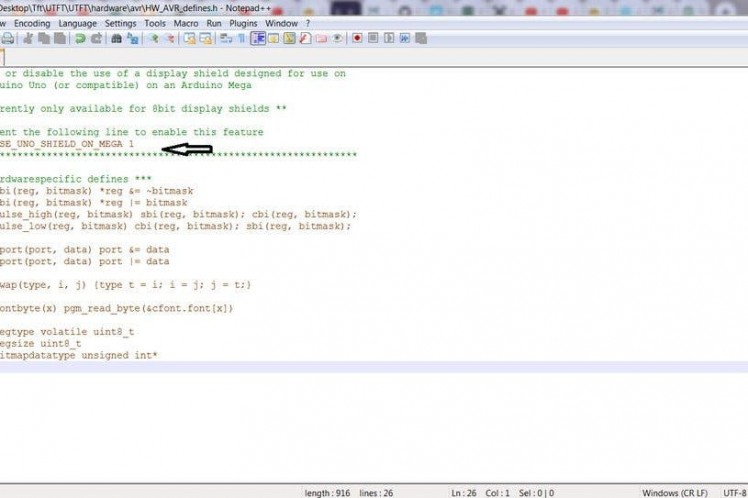
This library is the continuation of my ITDB02_Graph, ITDB02_Graph16 and RGB_GLCD libraries for Arduino and chipKit. As the number of supported display modules and controllers started to increase I felt it was time to make a single, universal library as it will be much easier to maintain in the future.
Arduino MEGA has 256kb of program memory. In addition, there are 54 pins.
Most of them are free to use, and the analog just 5 are taken from 16.
This library supports a number of 8bit, 16bit, and serial graphics displays, and will work with both Arduino, chipKit boards and select TI LaunchPads.
NOTE:Due to the size of the library I do not recommend using it on ATmega328 (Arduino Uno) and ATmega32U4 (Arduino Leonardo) as they only have 32KB of flash memory. It will work, but you will be severely limited in available flash memory for your application.
Steps:
- Download the UTFT Library
- Unzip the Library
- Open UTFThardwareavr in case of Arduino or depending on the microcontroller used
- Open HW_AVR_defines using Notepad
- Uncomment Line 7 to enable UNO shield for MEGA
- Save the file and Add this Library to Arduino IDE
Now we are done with this step! In the next step, I'll show to use the library and define the pins for Arduino Mega.
Step 3: Initializing the TFT Shield
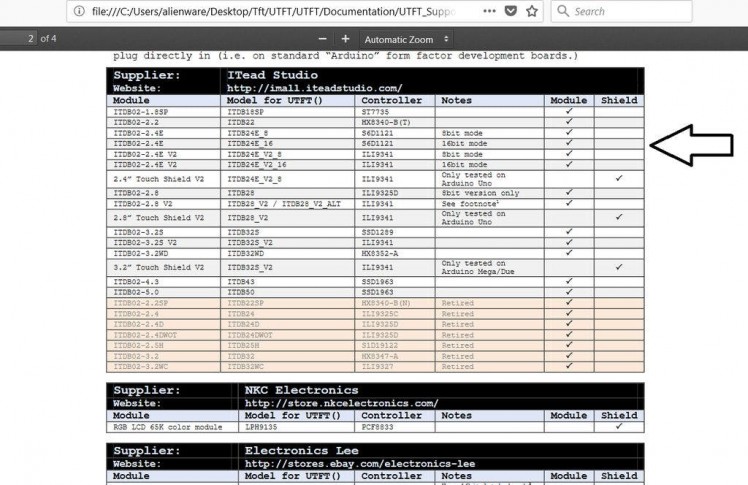
- After editing the library, Add it to the Arduino directory.
Next, I'm gonna show you how to define the right TFT module that you have
we should find its module name in the library.
- open the library file
- go to the documentation
You can see these files in the Documentation
• UTFT:
This file shows all the functions and commands that included in this library.
• UTFT_Requirement
This file has the information about the modules and how it's related to the library, like pins configurations
•UTFT_Supported_display_modules_&_controller
This is our target, this file has the names of the modules and shields that are supported by this library, you can see in it a list of module names and module names for the UTFT which you should use to define your module.
Steps to Define the TFT:
Open UTFT_Supported_display_modules_&_controller file from the library
- Open UTFT_Supported_display_modules_&_controller file from the library
- Find the Models for UTFT for the Modules (shield) which you have.
- Now to define a UTFT function on the Arduino IDE, we use the command :
UTFT name(module, Rs, Wr, Cs, Rst);
Open UTFT_Requirement file from the library
From the document, we know that the pins are located on the A5, A4, A3, and A2 pins.
we use the command:
UTFT myGLCD(ITDB28, 19, 18, 17, 16); # note that pins 19, 18, 17, 16 in the Arduino Mega
UTFT myGLCD(ITDB28, A5, A4, A3, A2); # note that pins A5, A4, A3, A2 in the Arduino UNO
And done! Now you can use the library examples on the Arduino IDE with the Following changes.
Step 4: Basic Hello World
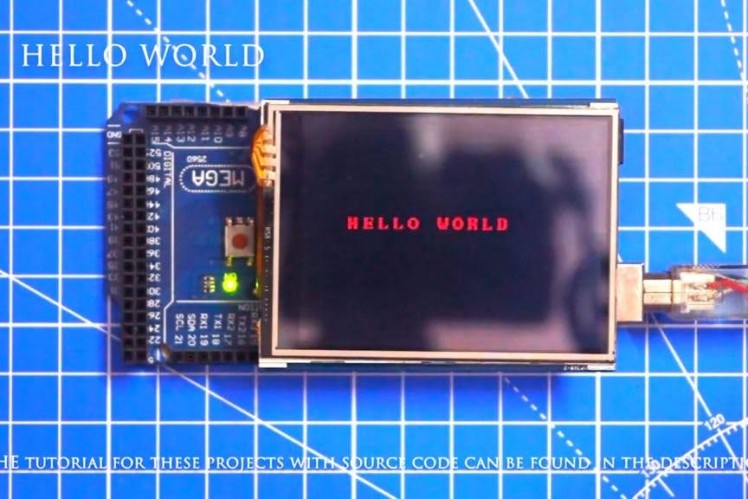
#include <UTFT.h>
// Declare which fonts we will be using
extern uint8_t BigFont[];
extern uint8_t SevenSegNumFont[];
// Remember to change the model parameter to suit your display module!
UTFT myGLCD(ITDB28, A5, A4, A3, A2);
void setup()
{
myGLCD.InitLCD();
myGLCD.clrScr();
myGLCD.setFont(BigFont);
}
void loop()
{
myGLCD.setColor(0, 255, 0); //green
myGLCD.print("HELLO WORLD", 45, 100);
while (true) {};
}
Step 5: UTFT Fonts
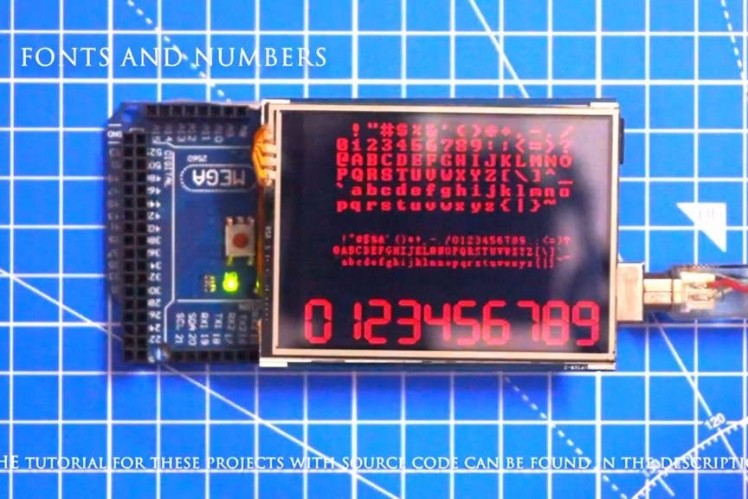
#include <UTFT.h>
// Declare which fonts we will be using
extern uint8_t SmallFont[];
extern uint8_t BigFont[];
extern uint8_t SevenSegNumFont[];
// Set the pins to the correct ones for your development shield
// ------------------------------------------------------------
// Arduino Uno / 2009:
// -------------------
// Standard Arduino Uno/2009 shield : <display model>,A5,A4,A3,A2
// DisplayModule Arduino Uno TFT shield : <display model>,A5,A4,A3,A2
//
// Arduino Mega:
// -------------------
// Standard Arduino Mega/Due shield : <display model>,38,39,40,41
// CTE TFT LCD/SD Shield for Arduino Mega : <display model>,38,39,40,41
//
// Remember to change the model parameter to suit your display module!
UTFT myGLCD(ITDB32S,38,39,40,41);
void setup()
{
myGLCD.InitLCD();
myGLCD.clrScr();
}
void loop()
{
myGLCD.setColor(0, 255, 0);
myGLCD.setBackColor(0, 0, 0);
myGLCD.setFont(BigFont);
myGLCD.print(" !"#$%&'()*+,-./", CENTER, 0);
myGLCD.print("0123456789:;<=>?", CENTER, 16);
myGLCD.print("@ABCDEFGHIJKLMNO", CENTER, 32);
myGLCD.print("PQRSTUVWXYZ[\]^_", CENTER, 48);
myGLCD.print("`abcdefghijklmno", CENTER, 64);
myGLCD.print("pqrstuvwxyz{|}~ ", CENTER, 80);
myGLCD.setFont(SmallFont);
myGLCD.print(" !"#$%&'()*+,-./0123456789:;<=>?", CENTER, 120);
myGLCD.print("@ABCDEFGHIJKLMNOPQRSTUVWXYZ[\]^_", CENTER, 132);
myGLCD.print("`abcdefghijklmnopqrstuvwxyz{|}~ ", CENTER, 144);
myGLCD.setFont(SevenSegNumFont);
myGLCD.print("0123456789", CENTER, 190);
while(1) {};
}
Step 6: UTFT Shapes, Lines and Pattern
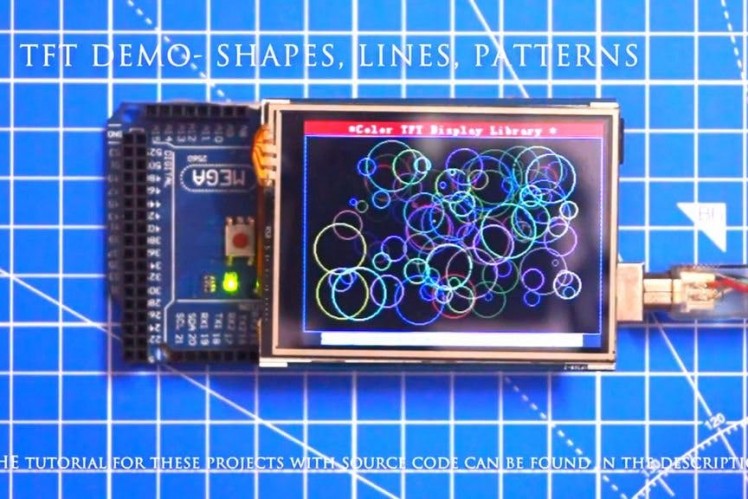
#include <UTFT.h>
// Declare which fonts we will be using
extern uint8_t SmallFont[];
// Set the pins to the correct ones for your development shield
// ------------------------------------------------------------
// Arduino Uno / 2009:
// -------------------
// Standard Arduino Uno/2009 shield : <display model>,A5,A4,A3,A2
// DisplayModule Arduino Uno TFT shield : <display model>,A5,A4,A3,A2
//
// Arduino Mega:
// -------------------
// Standard Arduino Mega/Due shield : <display model>,38,39,40,41
// CTE TFT LCD/SD Shield for Arduino Mega : <display model>,38,39,40,41
//
// Remember to change the model parameter to suit your display module!
UTFT myGLCD(ITDB32S,38,39,40,41);
void setup()
{
randomSeed(analogRead(0));
// Setup the LCD
myGLCD.InitLCD();
myGLCD.setFont(SmallFont);
}
void loop()
{
int buf[318];
int x, x2;
int y, y2;
int r;
// Clear the screen and draw the frame
myGLCD.clrScr();
myGLCD.setColor(255, 0, 0);
myGLCD.fillRect(0, 0, 319, 13);
myGLCD.setColor(64, 64, 64);
myGLCD.fillRect(0, 226, 319, 239);
myGLCD.setColor(255, 255, 255);
myGLCD.setBackColor(255, 0, 0);
myGLCD.print("* Universal Color TFT Display Library *", CENTER, 1);
myGLCD.setBackColor(64, 64, 64);
myGLCD.setColor(255,255,0);
myGLCD.print("<http://www.RinkyDinkElectronics.com/>", CENTER, 227);
myGLCD.setColor(0, 0, 255);
myGLCD.drawRect(0, 14, 319, 225);
// Draw crosshairs
myGLCD.setColor(0, 0, 255);
myGLCD.setBackColor(0, 0, 0);
myGLCD.drawLine(159, 15, 159, 224);
myGLCD.drawLine(1, 119, 318, 119);
for (int i=9; i<310; i+=10)
myGLCD.drawLine(i, 117, i, 121);
for (int i=19; i<220; i+=10)
myGLCD.drawLine(157, i, 161, i);
// Draw sin-, cos- and tan-lines
myGLCD.setColor(0,255,255);
myGLCD.print("Sin", 5, 15);
for (int i=1; i<318; i++)
{
myGLCD.drawPixel(i,119+(sin(((i*1.13)*3.14)/180)*95));
}
myGLCD.setColor(255,0,0);
myGLCD.print("Cos", 5, 27);
for (int i=1; i<318; i++)
{
myGLCD.drawPixel(i,119+(cos(((i*1.13)*3.14)/180)*95));
}
myGLCD.setColor(255,255,0);
myGLCD.print("Tan", 5, 39);
for (int i=1; i<318; i++)
{
myGLCD.drawPixel(i,119+(tan(((i*1.13)*3.14)/180)));
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
myGLCD.setColor(0, 0, 255);
myGLCD.setBackColor(0, 0, 0);
myGLCD.drawLine(159, 15, 159, 224);
myGLCD.drawLine(1, 119, 318, 119);
// Draw a moving sinewave
x=1;
for (int i=1; i<(318*20); i++)
{
x++;
if (x==319)
x=1;
if (i>319)
{
if ((x==159)||(buf[x-1]==119))
myGLCD.setColor(0,0,255);
else
myGLCD.setColor(0,0,0);
myGLCD.drawPixel(x,buf[x-1]);
}
myGLCD.setColor(0,255,255);
y=119+(sin(((i*1.1)*3.14)/180)*(90-(i / 100)));
myGLCD.drawPixel(x,y);
buf[x-1]=y;
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
// Draw some filled rectangles
for (int i=1; i<6; i++)
{
switch (i)
{
case 1:
myGLCD.setColor(255,0,255);
break;
case 2:
myGLCD.setColor(255,0,0);
break;
case 3:
myGLCD.setColor(0,255,0);
break;
case 4:
myGLCD.setColor(0,0,255);
break;
case 5:
myGLCD.setColor(255,255,0);
break;
}
myGLCD.fillRect(70+(i*20), 30+(i*20), 130+(i*20), 90+(i*20));
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
// Draw some filled, rounded rectangles
for (int i=1; i<6; i++)
{
switch (i)
{
case 1:
myGLCD.setColor(255,0,255);
break;
case 2:
myGLCD.setColor(255,0,0);
break;
case 3:
myGLCD.setColor(0,255,0);
break;
case 4:
myGLCD.setColor(0,0,255);
break;
case 5:
myGLCD.setColor(255,255,0);
break;
}
myGLCD.fillRoundRect(190-(i*20), 30+(i*20), 250-(i*20), 90+(i*20));
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
// Draw some filled circles
for (int i=1; i<6; i++)
{
switch (i)
{
case 1:
myGLCD.setColor(255,0,255);
break;
case 2:
myGLCD.setColor(255,0,0);
break;
case 3:
myGLCD.setColor(0,255,0);
break;
case 4:
myGLCD.setColor(0,0,255);
break;
case 5:
myGLCD.setColor(255,255,0);
break;
}
myGLCD.fillCircle(100+(i*20),60+(i*20), 30);
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
// Draw some lines in a pattern
myGLCD.setColor (255,0,0);
for (int i=15; i<224; i+=5)
{
myGLCD.drawLine(1, i, (i*1.44)-10, 224);
}
myGLCD.setColor (255,0,0);
for (int i=224; i>15; i-=5)
{
myGLCD.drawLine(318, i, (i*1.44)-11, 15);
}
myGLCD.setColor (0,255,255);
for (int i=224; i>15; i-=5)
{
myGLCD.drawLine(1, i, 331-(i*1.44), 15);
}
myGLCD.setColor (0,255,255);
for (int i=15; i<224; i+=5)
{
myGLCD.drawLine(318, i, 330-(i*1.44), 224);
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
// Draw some random circles
for (int i=0; i<100; i++)
{
myGLCD.setColor(random(255), random(255), random(255));
x=32+random(256);
y=45+random(146);
r=random(30);
myGLCD.drawCircle(x, y, r);
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
// Draw some random rectangles
for (int i=0; i<100; i++)
{
myGLCD.setColor(random(255), random(255), random(255));
x=2+random(316);
y=16+random(207);
x2=2+random(316);
y2=16+random(207);
myGLCD.drawRect(x, y, x2, y2);
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
// Draw some random rounded rectangles
for (int i=0; i<100; i++)
{
myGLCD.setColor(random(255), random(255), random(255));
x=2+random(316);
y=16+random(207);
x2=2+random(316);
y2=16+random(207);
myGLCD.drawRoundRect(x, y, x2, y2);
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
for (int i=0; i<100; i++)
{
myGLCD.setColor(random(255), random(255), random(255));
x=2+random(316);
y=16+random(209);
x2=2+random(316);
y2=16+random(209);
myGLCD.drawLine(x, y, x2, y2);
}
delay(2000);
myGLCD.setColor(0,0,0);
myGLCD.fillRect(1,15,318,224);
for (int i=0; i<10000; i++)
{
myGLCD.setColor(random(255), random(255), random(255));
myGLCD.drawPixel(2+random(316), 16+random(209));
}
delay(2000);
myGLCD.fillScr(0, 0, 255);
myGLCD.setColor(255, 0, 0);
myGLCD.fillRoundRect(80, 70, 239, 169);
myGLCD.setColor(255, 255, 255);
myGLCD.setBackColor(255, 0, 0);
myGLCD.print("That's it!", CENTER, 93);
myGLCD.print("Restarting in a", CENTER, 119);
myGLCD.print("few seconds...", CENTER, 132);
myGLCD.setColor(0, 255, 0);
myGLCD.setBackColor(0, 0, 255);
myGLCD.print("Runtime: (msecs)", CENTER, 210);
myGLCD.printNumI(millis(), CENTER, 225);
delay (10000);
}
Step 7: UTFT Bitmap
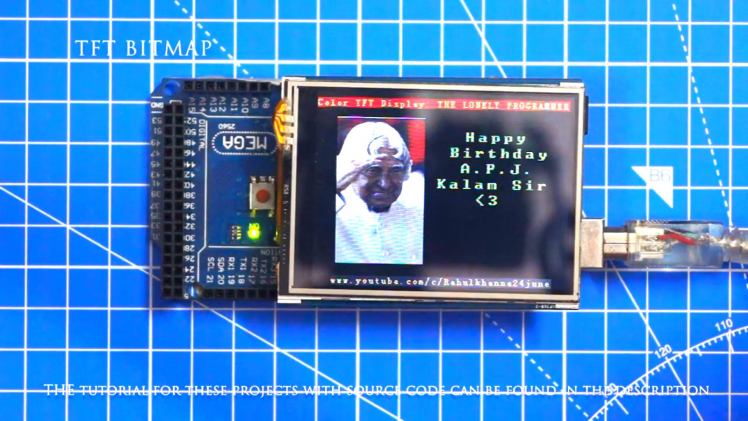
#include <UTFT.h>
#include <avr/pgmspace.h>
// Declare which fonts we will be using
extern uint8_t SmallFont[];
// Set the pins to the correct ones for your development shield
// ------------------------------------------------------------
// Arduino Uno / 2009:
// -------------------
// Standard Arduino Uno/2009 shield : <display model>,A5,A4,A3,A2
// DisplayModule Arduino Uno TFT shield : <display model>,A5,A4,A3,A2
//
// Arduino Mega:
// -------------------
// Standard Arduino Mega/Due shield : <display model>,38,39,40,41
// CTE TFT LCD/SD Shield for Arduino Mega : <display model>,38,39,40,41
//
// Remember to change the model parameter to suit your display module!
UTFT myGLCD(ITDB32S,A5,A4,A3,A2);
extern unsigned int info[0x400];
extern unsigned int icon[0x400];
extern unsigned int tux[0x400];
void setup()
{
myGLCD.InitLCD();
myGLCD.setFont(SmallFont);
}
void loop()
{
myGLCD.fillScr(255, 255, 255);
myGLCD.setColor(255, 255, 255);
myGLCD.print(" *** A 10 by 7 grid of a 32x32 icon *** ", CENTER, 228);
for (int x=0; x<10; x++)
for (int y=0; y<7; y++)
myGLCD.drawBitmap (x*32, y*32, 32, 32, info);
delay(5000);
myGLCD.fillScr(255, 255, 255);
myGLCD.setColor(255, 255, 255);
myGLCD.print(" Two different icons in scale 1 to 4 ", CENTER, 228);
int x=0;
for (int s=0; s<4; s++)
{
x+=(s*32);
myGLCD.drawBitmap (x, 0, 32, 32, tux, s+1);
}
x=0;
for (int s=4; s>0; s--)
{
myGLCD.drawBitmap (x, 224-(s*32), 32, 32, icon, s);
x+=(s*32);
}
delay(5000);
}
Step 8: Button Interfacing
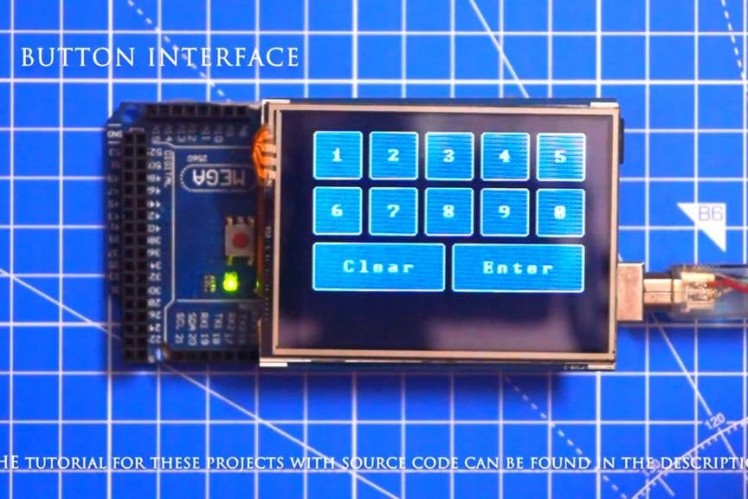
#include <UTFT.h>
#include <URTouch.h>
// Initialize display
// ------------------
// Set the pins to the correct ones for your development board
// -----------------------------------------------------------
// Standard Arduino Uno/2009 Shield : <display model>,19,18,17,16
// Standard Arduino Mega/Due shield : <display model>,38,39,40,41
// CTE TFT LCD/SD Shield for Arduino Due : <display model>,25,26,27,28
// Teensy 3.x TFT Test Board : <display model>,23,22, 3, 4
// ElecHouse TFT LCD/SD Shield for Arduino Due : <display model>,22,23,31,33
//
// Remember to change the model parameter to suit your display module!
UTFT myGLCD(ITDB32S,38,39,40,41);
// Initialize touchscreen
// ----------------------
// Set the pins to the correct ones for your development board
// -----------------------------------------------------------
// Standard Arduino Uno/2009 Shield : 15,10,14, 9, 8
// Standard Arduino Mega/Due shield : 6, 5, 4, 3, 2
// CTE TFT LCD/SD Shield for Arduino Due : 6, 5, 4, 3, 2
// Teensy 3.x TFT Test Board : 26,31,27,28,29
// ElecHouse TFT LCD/SD Shield for Arduino Due : 25,26,27,29,30
//
URTouch myTouch( 6, 5, 4, 3, 2);
// Declare which fonts we will be using
extern uint8_t BigFont[];
int x, y;
char stCurrent[20]="";
int stCurrentLen=0;
char stLast[20]="";
/*************************
** Custom functions **
*************************/
void drawButtons()
{
// Draw the upper row of buttons
for (x=0; x<5; x++)
{
myGLCD.setColor(0, 0, 255);
myGLCD.fillRoundRect (10+(x*60), 10, 60+(x*60), 60);
myGLCD.setColor(255, 255, 255);
myGLCD.drawRoundRect (10+(x*60), 10, 60+(x*60), 60);
myGLCD.printNumI(x+1, 27+(x*60), 27);
}
// Draw the center row of buttons
for (x=0; x<5; x++)
{
myGLCD.setColor(0, 0, 255);
myGLCD.fillRoundRect (10+(x*60), 70, 60+(x*60), 120);
myGLCD.setColor(255, 255, 255);
myGLCD.drawRoundRect (10+(x*60), 70, 60+(x*60), 120);
if (x<4)
myGLCD.printNumI(x+6, 27+(x*60), 87);
}
myGLCD.print("0", 267, 87);
// Draw the lower row of buttons
myGLCD.setColor(0, 0, 255);
myGLCD.fillRoundRect (10, 130, 150, 180);
myGLCD.setColor(255, 255, 255);
myGLCD.drawRoundRect (10, 130, 150, 180);
myGLCD.print("Clear", 40, 147);
myGLCD.setColor(0, 0, 255);
myGLCD.fillRoundRect (160, 130, 300, 180);
myGLCD.setColor(255, 255, 255);
myGLCD.drawRoundRect (160, 130, 300, 180);
myGLCD.print("Enter", 190, 147);
myGLCD.setBackColor (0, 0, 0);
}
void updateStr(int val)
{
if (stCurrentLen<20)
{
stCurrent[stCurrentLen]=val;
stCurrent[stCurrentLen+1]='
Schematics, diagrams and documents
Code
Credits
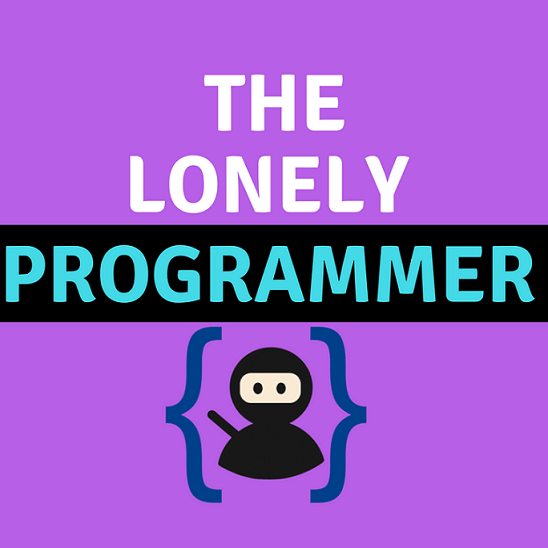
the lonely programmer
Passionate Techie ! Robotics | Electronics | Programming Hey Geek! If you are in search of electronics projects, Arduino based projects or any Micro-controller based projects, this channel is for you. In this channel, we build electronics projects using the impressive and low-cost boards that are available today. If you are a maker or if you want to learn how to make your own Arduino projects and other interesting Robots, do subscribe the channel to be a part of this community. We develop our own hardware and software projects and will try to build something new. Don’t worry if you don’t know how to program. I'll share the algorithm if you face any difficulties.
Leave your feedback...