Puck.js With Sms Control
About the project
This video shows you how use Puck.js and a GSM modem to control devices via SMS text messages.
Project info
Difficulty: Easy
Platforms: Espruino
Estimated time: 2 hours
License: Apache License 2.0 (Apache-2.0)
Items used in this project
Hardware components
Story
This video shows you how use Puck.js and a GSM modem to control devices via SMS text messages.
The video further down shows how to read data from BLE devices within range and then send that as an SMS text message response.
Note: You'll need the latest firmware as of Sept 2017 on your Puck.js device for this. That means at least 1v95 (when released) or a 'cutting edge' build (http://www.espruino.com/Download).
Wiring Up
Connect as follows:
Software
Controlling Puck.js's LED from an SMS
- Bluetooth.setConsole(1);
- Serial1.setup(115200, { rx: D29, tx : D28 });
- var ATSMS = require("ATSMS");
- var sms = new ATSMS(Serial1);
- sms.init(function(err) {
- if (err) throw err;
- console.log("Initialised!");
- sms.list("ALL", function(err,list) {
- if (err) throw err;
- if (list.length)
- console.log(list);
- else
- console.log("No Messages");
- });
- // and to send a message:
- //sms.send('+441234567890','Hello world!', callback)
- });
- sms.on('message', function(msg) {
- console.log("Got message #",msg);
- sms.get(msg, function(err, msg) {
- if (err) throw err;
- print("Read message", msg);
- var txt = msg.text.toLowerCase();
- if (txt=="on") LED1.set();
- if (txt=="off") LED1.reset();
- // delete all messages to stop us overflowing
- sms.delete("ALL");
- });
- });
Controlling an Awox BLE Lightbulb
- Bluetooth.setConsole(1);
- Serial1.setup(115200, { rx: D29, tx : D28 });
- var ATSMS = require("ATSMS");
- var sms = new ATSMS(Serial1);
- sms.init(function(err) {
- if (err) throw err;
- console.log("Initialised!");
- sms.list("ALL", function(err,list) {
- if (err) throw err;
- if (list.length)
- console.log(list);
- else
- console.log("No Messages");
- });
- // and to send a message:
- //sms.send('+441234567890','Hello world!', callback)
- });
- sms.on('message', function(msg) {
- console.log("Got message #",msg);
- sms.get(msg, function(err, msg) {
- if (err) throw err;
- print("Read message");
- var txt = msg.text.toLowerCase();
- if (txt=="on") setLight(1);
- if (txt=="off") setLight(0);
- // delete all messages to stop us overflowing
- sms.delete("ALL");
- });
- });
- function setLight(isOn) {
- var gatt;
- NRF.connect("98:7b:f3:61:1c:22").then(function(g) {
- // ^^^^^^^^^^^^^^^^^ your light's address here
- gatt = g;
- return gatt.getPrimaryService("33160fb9-5b27-4e70-b0f8-ff411e3ae078");
- }).then(function(service) {
- return service.getCharacteristic("217887f8-0af2-4002-9c05-24c9ecf71600");
- }).then(function(characteristic) {
- return characteristic.writeValue(isOn ? 1 : 0);
- }).then(function() {
- gatt.disconnect();
- console.log("Done!");
- });
- }
Returning temperature
The code on the Puck.js sending the temperature is:
- setInterval(function() {
- NRF.setAdvertising({
- 0x1809 : [Math.round(E.getTemperature())]
- });
- }, 30000);
and the full source is:
- Bluetooth.setConsole(1);
- Serial1.setup(115200, { rx: D29, tx : D28 });
- var ATSMS = require("ATSMS");
- var sms = new ATSMS(Serial1);
- sms.init(function(err) {
- if (err) throw err;
- console.log("Initialised!");
- sms.list("ALL", function(err,list) {
- if (err) throw err;
- if (list.length)
- console.log(list);
- else
- console.log("No Messages");
- });
- // and to send a message:
- //sms.send('+441234567890','Hello world!', callback)
- });
- sms.on('message', function(msg) {
- console.log("Got message #",msg);
- sms.get(msg, function(err, msg) {
- if (err) throw err;
- print("Read message", msg);
- var txt = msg.text.toLowerCase();
- if (txt=="on") LED1.set();
- if (txt=="off") LED1.reset();
- if (txt=="get") getTemp(msg.oaddr);
- // delete all messages to stop us overflowing
- sms.delete("ALL");
- });
- });
- function getTemp(number) {
- console.log("Getting temp");
- NRF.findDevices(function(devs) {
- devs.forEach(function(dev) {
- if (dev.name=="Puck.js 5736") { // <--- change this to the name of your Puck.js
- console.log("Got temp");
- var message = "Temp is "+dev.serviceData["1809"][0];
- sms.send(number,message, function() {
- print("Sent text!");
- });
- }
- });
- });
- }
Buying
See the Puck.js Lightbulb page for links to where to find the Awox lights for sale.
The SIM900 page has links to where to find the SIM900 module.
Puck.js devices can be ordered from here
Credits
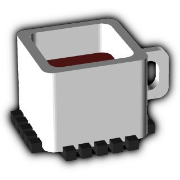
Espruino
Espruino, Espruino Pico and Puck.js are low-power Microcontrollers that run JavaScript. Espruino is a JavaScript Interpreter for Microcontrollers that is designed to make development quick and easy. The Espruino interpreter is firmware that runs on a variety of different microcontrollers, but we also make Espruino Boards that come with the interpreter pre-installed and are the easiest devices to get started with. However Espruino itself isn't just the interpreter firmware or hardware - there's also the Web IDE, command-line tools, documentation, tutorials, and modules that form a complete solution for embedded software development.
Leave your feedback...