Sense Hat Random Sparkles
About the project
Create amazing random sparkles with your Sense HAT. In doing so, you will learn how to choose random positions on the Sense HAT’s LED display as well as random colours to light up the LEDs.
Project info
Difficulty: Moderate
Platforms: Raspberry Pi
Estimated time: 1 day
License: Creative Commons Attribution CC BY version 4.0 or later (CC BY 4+)
Items used in this project
Hardware components
Software apps and online services
Story
If you do not have a Raspberry Pi, you can create the project using:
- Online Sense HAT emulator on Trinket
Software
If you’re using a Raspberry Pi, you will need the latest version of Raspbian, which already includes the following software packages:
- Python 3
- Sense HAT module for Python 3
If for any reason you need to install a package manually, follow these instructions:
Install a software package on the Raspberry Pi
Your Raspberry Pi will need to be online to install packages. Before installing a package, update and upgrade Raspbian, your Raspberry Pi’s operating system.
- Open a terminal window and enter the following commands to do this:
- sudo apt-get update
- sudo apt-get upgrade
- Now you can install the packages you’ll need by typing install commands into the terminal window. For example, here’s how to install the Sense HAT software:
- sudo apt-get install sense-hat
Type this command into a terminal window to install the Sense HAT package:
- sudo apt-get install sense-hat
Connect to your Sense HAT
If you have a Sense HAT, attach it to your Raspberry Pi.
Attaching a Sense HAT
Before attaching any HAT to your Raspberry Pi, ensure that the Pi is shut down.
- Remove the Sense HAT and parts from their packaging.
- Use two of the provided screws to attach the spacers to your Raspberry Pi, as shown below.
Note: the above step is optional — you do not have to attach the standoffs to the Sense HAT for it to work.
- Then push the Sense HAT carefully onto the pins of your Raspberry Pi, and secure it with the remaining screws.
Note: using a metal stand-off next to the Raspberry Pi 3’s wireless antenna will degrade its performance and range. Either leave out this stand-off, or use nylon stand-offs and nylon screws instead.
Pro tip: be careful when taking off the Sense HAT, as the 40-pin black header tends to get stuck.
- If you do not have a Sense HAT, you could create the project using the Sense HAT emulator.
Using the Sense HAT emulator
If you don’t have access to a Sense HAT, you can use the emulator.
Online Sense HAT emulator
There is an online emulator you can use in your browser to write and test code for the Sense HAT.
- Open an internet browser, go to https://trinket.io/sense-hat and delete the existing demo code which is in the editor.
- If you would like to save your work, you will need to create a free account on the Trinket website.
Sense HAT emulator on the Raspberry Pi
If you are using a Raspberry Pi, there is a Sense HAT emulator included in the Raspbian operating system.
- From the main menu, select Programming > Sense HAT emulator to open a window containing the emulator.
- If you are using this version of the emulator, your program must import from sense_emu instead of sense_hat:
- from sense_emu import SenseHat
If you later want to run your code on a real Sense HAT, just change the import line as shown below. All other code can remain exactly the same.
- from sense_hat import SenseHat
Note: if you are using a Sense HAT, you will be using IDLE for your code. If you are using the Sense HAT emulator, you will be using Trinket. Depending on which option you are using, there may be some differences in the colour of the text highlighting.
Setting pixels at random
First, we’ll think up some random numbers and use the set_pixel function to place a random colour on a random location on the Sense HAT display.
- Open the IDLE editor.
Opening IDLE3
IDLE is Python’s Integrated Development Environment, which you can use to write and run code.
To open IDLE, go to the menu and choose Programming.
You should see two versions of IDLE - make sure you click on the one that says .
To create a new file in IDLE, you can click on File and then New File in IDLE’s menu bar.This will open a second window in which you can write your code.
- Create a new file and save it as sparkles.py.
- In the new file, start by importing the SenseHat module:
- from sense_hat import SenseHat
- Next, create a connection to your Sense HAT by adding this line of code:
- sense = SenseHat()
We will then define x and y, to choose which pixel on the Sense HAT will light.
- Create a variable called x, and set it equal to a number of your choice between 0 and 7. This will be the x coordinate of your pixel on the display.
Creating a variable in Python
A variable allows you to store data within a program. Variables have a name and a value. This variable has the name animal and the value cat:
animal = "cat"
This variable has the name score and the value 30:
score = 30
To create a variable, give it a name and set it equal to a value. The name of the variable always goes on the left, so this code is wrong:
# This code is wrong
30 = score
- Create another variable called y, and set it equal to another number between 0 and 7. This will be the y coordinate of your pixel on the display.
- To choose the colour of your pixel, think of three numbers between 0 and 255, then assign them to variables called r, g, and b. These variables will represent the colour of your pixel as amounts of red (r), green (g), and blue (b).
- Now use the set_pixel function to place a pixel with your randomly chosen colour at your randomly chosen location on the display.
Note: the collapsible directions below use a different filename than yours, and uses Trinket instead of IDLE.
Setting a single pixel on the Sense HAT
You can use the set_pixel command to control individual LEDs on the Sense HAT. To do this, you set the x and y variables the set_pixel command takes. x indicates the HAT’s horizontal axis, and can have a value between 0 (on the left) and 7 (on the right). y indicates the HAT’s vertical axis, and can have a value between 0 (at the ltop) and 7 (at the bottom). Therefore, the x, y coordinates 0, 0 address the top left-hand LED, and the x, y coordinates 7, 7 address the bottom right-hand LED.
The grid above corresponds with the Raspberry Pi when it is this way around:
Let try out this example for setting a different colour in each corner of the Sense HAT’s LED matrix. You will need to use the set_pixel command multiple times in your code, like this:
- from sense_hat import SenseHat
- sense = SenseHat() # This clears any pixels left on the Sense HAT. You may not need this step and may want to choose when to add it in.
- sense.clear()
- sense.set_pixel(0, 0, 255, 0, 0)
- sense.set_pixel(0, 7, 0, 255, 0)
- sense.set_pixel(7, 0, 0, 0, 255)
- sense.set_pixel(7, 7, 255, 0, 255)
Test setting the colour of different pixels using the Sense HAT Emulator:
The set_pixel method takes data in the following order:
x coordinate, y coordinate, red, green, blue
To define your set_pixel method, plug the names of your variables into the question marks in this line of code, in the right order: x coordinate, y coordinate, red, green, blue.
- sense.set_pixel(?, ?, ?, ?, ?)
View the hint below if you are stuck.
I need a hint
Here is how your finished code should look — you will probably have chosen different numbers:
- Run your code by pressing F5. You should see a single LED light up on the Sense HAT’s LED display.
- Now change all of the numbers in your program and run the program again. A second LED should turn on.
Using the random module
So far you picked your own random numbers, but you can let the computer choose them instead by using the random module.
- Add another import line at the top of your program:
- from random import randint
- Change your x and y variables to be equal to a random number between 0 and 7. Now your program will automatically select a random position on the LED matrix.
Randomness in Python
One of the standard modules in Python is the random module. You can use it to create pseudo-random numbers in your code.
randint
You can generate random integers between two values using the randint function. For example, the following line of code will produce a random integer between 0 and 10 (inclusive).
- from random import randint
- num = randint(0,10)
uniform
If you want a random floating-point number (also called float), you can use the uniform function. For example, the following line of code will produce a random float that’s equal to or greater than 0, but less than 10.
- from random import uniform
- num = uniform(0,10)
choice
If you want to choose a random item from a list, you can use the choice function.
- from random import choice
- deck = ['Ace', 'King', 'Queen', 'Jack']
- card = choice(deck)
- Run your program again, and you should see another random pixel being placed on the Sense HAT’s display. It will be the same colour you chose previously.
- Change your r, g, and b variables to each be equal to a random number between 0 and 255. Now your program will automatically select a random colour.
- Run the program again, and you should see another pixel appear in a random location, this time with a random colour.
- Run it a few more times, and you should see more of the grid fill up with random pixels.
If you have the sense.clear() line in your code, you will need to remove it. Otherwise, every time the program is re-run, the display will be cleared and your previous pixel will disappear.
Add a loop
Rather than running your program over and over by pressing F5, you can add a loop so that it will keep running by itself.
- You can use the sleep module to pause the program between pixels. To do so, first add another import to the top of your file.
- from time import sleep
- Add an infinite loop on the line below the import statements.
While True loop in Python
The purpose of a while loop is to repeat code over and over while a condition is True.
This is why while loops are sometimes referred to as condition-controlled loops.
The example below is a while loop that will run forever - an infinite loop. The loop will run forever because the condition is always True.
- while True:
- print("Hello world")
Note: The while line states the loop condition. The print line of code below it is slightly further to the right. This is called indentation - the line is indented to show that it is inside the loop. Any code inside the loop will be repeated.
An infinite loop is useful in situations where you want to perform the same actions over and over again, for example checking the value of a sensor. An infinite loop like this will continue to run forever meaning that any lines of code written after the loop will never happen. This is known as blocking - whereby a program blocks the execution of any other code.
- Indent all of the lines of code containing your variables and set_pixel so that they are within the loop:
I need a hint
- Add a line of code at the bottom of your program to pause for 0.1 seconds. Make sure that this line is indented level with the set_pixel line to show that it is inside the loop.
Using Python's sleep command
You can use the sleep function to temporarily pause your Python program.
- Add this line of code at the top of your program to import the sleep function.
- from time import sleep
- Whenever you want a pause in your program, call the sleep function. The number in the brackets indicates how many seconds you would like the pause to be.
- sleep(2)
You can pause for fractions of a second as well.
- sleep(0.5)
- Run the code, and you should see random sparkles in action!
Challenge: better sparkles
- Can you make the sparkles change more quickly?
- Can you make the sparkles appear in pastel colours? (Hint: you normally pick colour variable values within the range of 0 to 255. Reduce the range and see what happens.)
- Try fixing one of the colour values to 0, and see what happens.
- Try making the sparkles disappear again in a style of your choosing.
Credits
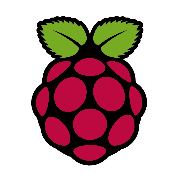
Raspberry Pi
Our mission is to put the power of computing and digital making into the hands of people all over the world. We do this so that more people are able to harness the power of computing and digital technologies for work, to solve problems that matter to them, and to express themselves creatively.
Leave your feedback...